Threads are the
independent units of execution and can run simultaneously with other threads.
Threads helps in achieving parallel execution in C#, which is also called
multithreading.
A C# program
typically starts with a single thread create automatically by CLR (Common
Language Runtime) and operating system. If needed, additional threads has to be
created manually through code.
An example of
multithreaded application is Microsoft Word (well known to all of us). When you
are typing in Microsoft word, an another thread is running in background which
performs spell check and highlights the misspelled words.
Threads are available in System.Threading namespace.
Following code
snippet shows simple example of thread or multi thread environment in C#.
using System; using System.Threading; namespace ThreadSample { class Program { static void Main(string[] args) { Thread t = new Thread(DoWork); // create a new thread t.Start(); // start new thread // Simultaneously, do work on the main thread. for (int i = 0; i < 100; i++) Console.Write("threaded "); Console.ReadKey(); } static void DoWork() { for (int i = 0; i < 100; i++) Console.Write("minds "); } } }Here is the output of the above program, you can see threaded and minds written simultaneously on the console.
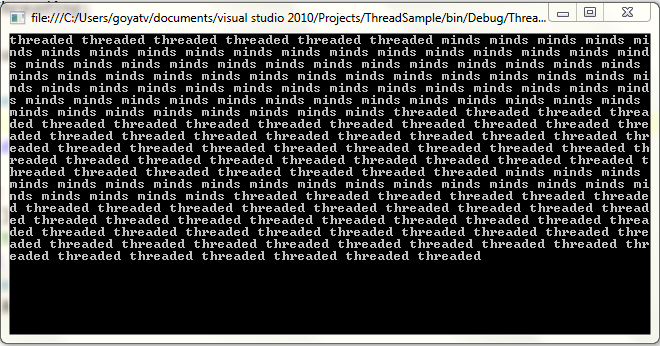
No comments :
Post a Comment
Threaded Minds would love to hear from you !!!