DateTime.Now is a built in property provided by .NET framework which returns the current time of the machine which is executing the code.
This property internally converts the DateTime.UtcNow provided value to the date and time format of the machine executing the code. Hence this property has an overhead of execution, so must be used carefully.
DateTime.UtcNow is another built in property provided by .NET framework which returns the current date and time in UTC. The value returned by this property is independent of the system executing the code.
Since DateTime.Now internally uses DateTime.UtcNow, DateTime.Now is a performance hit in applications where performance is a concern. If you have to do some calculations on the date and time data then I prefer to use DateTime.UtcNow, since it doesn't affect the calculation. In most case date and time are stored in database to display on the user interface, in such cases I suggest to store the date and time in UTC format and convert it to machine date time format while accessing the data.
Here is a simple piece of code which shows the execution time difference b/w DateTime.Now and DateTime.UtcNow.
Here is the graph showing the execution time comparison of both based upon output of above code.
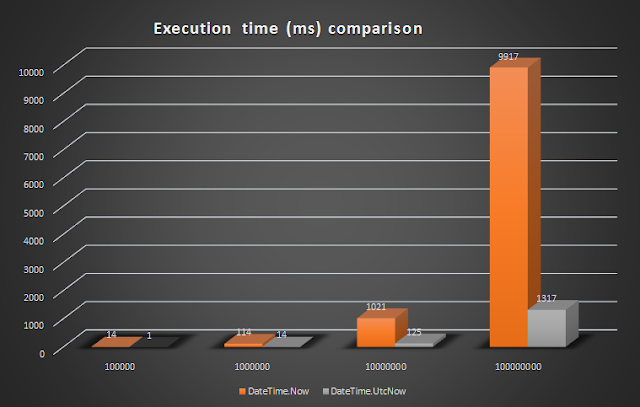
This property internally converts the DateTime.UtcNow provided value to the date and time format of the machine executing the code. Hence this property has an overhead of execution, so must be used carefully.
DateTime.UtcNow is another built in property provided by .NET framework which returns the current date and time in UTC. The value returned by this property is independent of the system executing the code.
Since DateTime.Now internally uses DateTime.UtcNow, DateTime.Now is a performance hit in applications where performance is a concern. If you have to do some calculations on the date and time data then I prefer to use DateTime.UtcNow, since it doesn't affect the calculation. In most case date and time are stored in database to display on the user interface, in such cases I suggest to store the date and time in UTC format and convert it to machine date time format while accessing the data.
using System; using System.Diagnostics; namespace DateTimeComp { class Program { private static readonly int[] ArrayCount = new int[] { 100000, 1000000, 10000000, 10000000, 1000000000 }; static void Main(string[] args) { foreach (int count in ArrayCount) { Console.WriteLine("***** {0} *****", count); Stopwatch watchNow = new Stopwatch(); watchNow.Start(); for (int i = 0; i < count; i++) { DateTime time = DateTime.Now; } watchNow.Stop(); Console.WriteLine("DateTime.Now : {0}", watchNow.ElapsedMilliseconds); Stopwatch watchUtcNow = new Stopwatch(); watchUtcNow.Start(); for (int i = 0; i < count; i++) { DateTime time = DateTime.UtcNow; } watchUtcNow.Stop(); Console.WriteLine("DateTime.UtcNow : {0}", watchUtcNow.ElapsedMilliseconds); //Console.WriteLine(string.Format("***** {0} *****", count)); } Console.ReadKey(); } } }
Here is the graph showing the execution time comparison of both based upon output of above code.
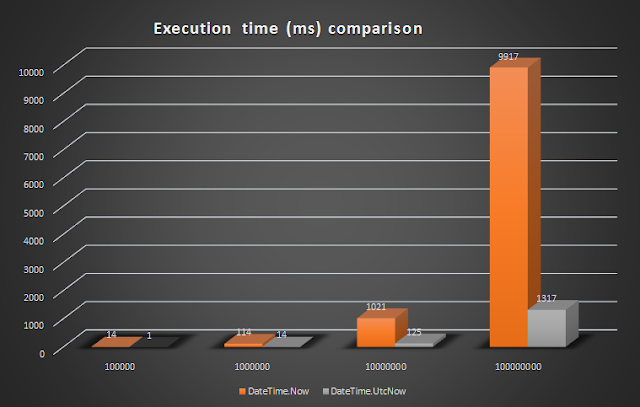
No comments :
Post a Comment
Threaded Minds would love to hear from you !!!